22 Unit Testing Frameworks and Their Pros and Cons
Developers often spend more time writing unit tests than writing the code they are testing. Most of the time, it is not because their code is bad but because they failed to pick a unit testing framework that fits the use case. The wrong framework means slow runtimes, painful syntax, or tests that constantly break with every minor refactor. Yet, most development teams still choose a testing framework the same way they pick pizza toppings out of habit and familiarity instead of strategy.
According to the Stack Overflow Developer Survey, over 75% of developers write unit tests, but fewer than 30% feel confident those tests actually prevent regressions.
So, if you have ever asked yourself, “Why are my tests so brittle?”, “Which unit testing framework should I use?” or “Why is test setup so painful?” you are not alone. Let us take you through the specifics of 22 widely adopted testing frameworks to make the framework selection less confusing.
Table of Contents
Choosing unit testing frameworks goes beyond picking up whatever is trending and involves optimizing for long-term velocity and reliability. Below are several evaluation criteria against which you can compare the tools:
- Language compatibility.
- Test speed, performance, and parallel execution capabilities.
- Ability to handle large codebases without slowing you down.
- Ease-of-setup, ease-of-use, and developer-friendly syntax.
- Integration with version control tools and CI/CD pipelines.
- Support for mocking, snapshot tests, and parameterization.
- Comprehensive test execution feedback and reporting.
- Community support, active maintenance, and plugins.
Choosing the right unit testing framework isn’t just about language support—it’s about how well it integrates with your tooling, how fast it runs, how readable your test output is, and how easy it is to maintain. Below is a breakdown of 22 frameworks, starting with those built for JavaScript and TypeScript. Each one comes with its own strengths, trade-offs, and gotchas. Pick what fits your stack and your workflow.
JavaScript’s testing ecosystem is crowded—some tools are built for speed, others for flexibility, and a few are just legacy holdovers that won’t die. Whether you're writing for Node, bundling with Webpack, managing a monorepo, or handling complex TypeScript test setups, these are the frameworks worth knowing.
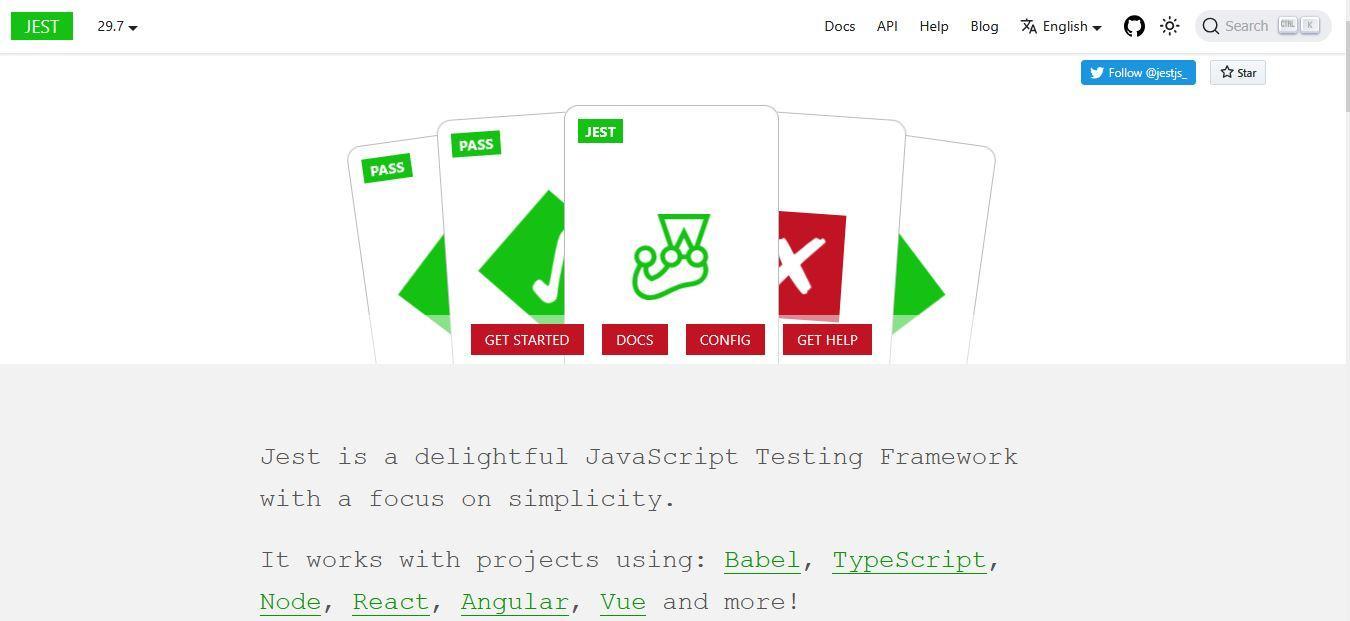
Best for: Scalable, all-in-one JavaScript and TypeScript testing in modern and legacy codebases.
Pros:
- Zero configuration, built-in snapshot testing and mocking, and parallel test execution for optimized performance.
- TypeScript support via ts-jest.
- Automatic mocking and comprehensive assertion library.
- Large community, widely adopted, and backed by Meta.
Cons:
- Can feel heavy for small projects.
- Performance hits in large monorepos.

Best for: Speed-optimized modern frontend testing (especially for projects using Vite)
Pros:
- Lightweight, performance-focused.
- Native ESM support.
- Smart and instant watch mode like HMR (hot module replacement) in Vite.
Cons:
- Vite-dependent and requires additional configurations to work with non-Vite projects like Webpack.
- Limited community, as it is in an early adoption phase.
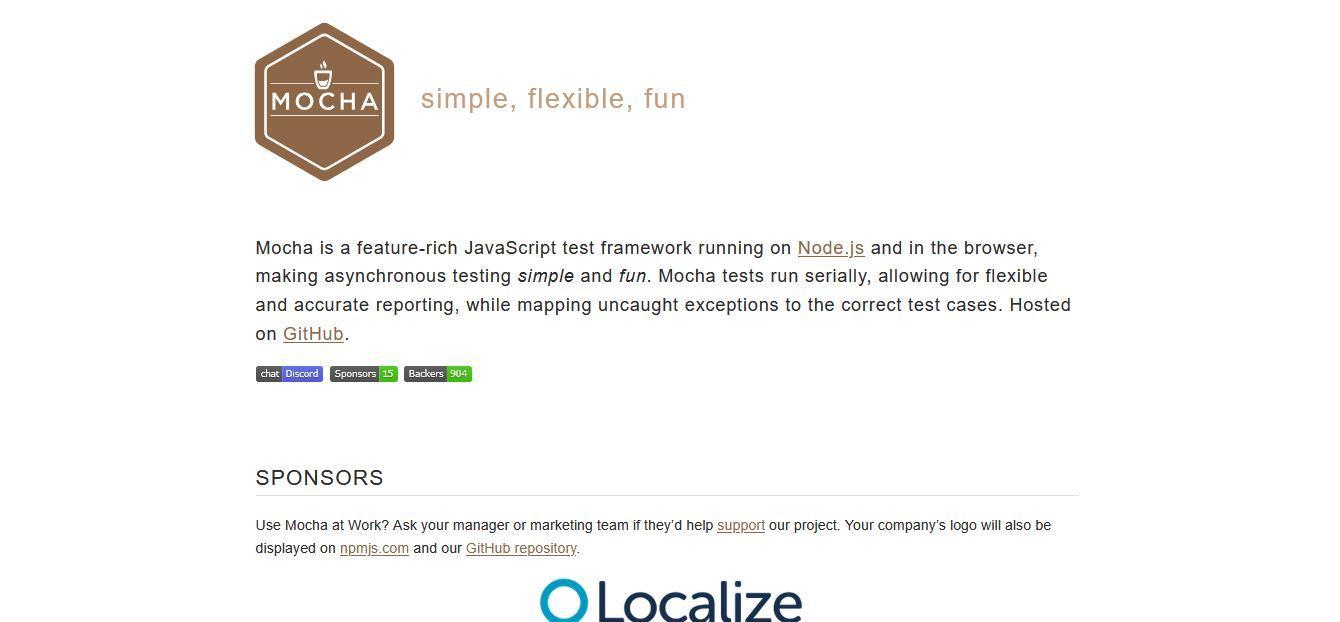
Best for: Highly customizable test setups
Pros:
- Supports synchronous and asynchronous testing.
- Supports both TDD (test-driven development) and BDD (behavior-driven development).
- Highly flexible and extensible.
Cons:
- Lacks built-in support for mocking, snapshots, and assertions, requiring assertion libraries like Chai.
- Complex configuration.
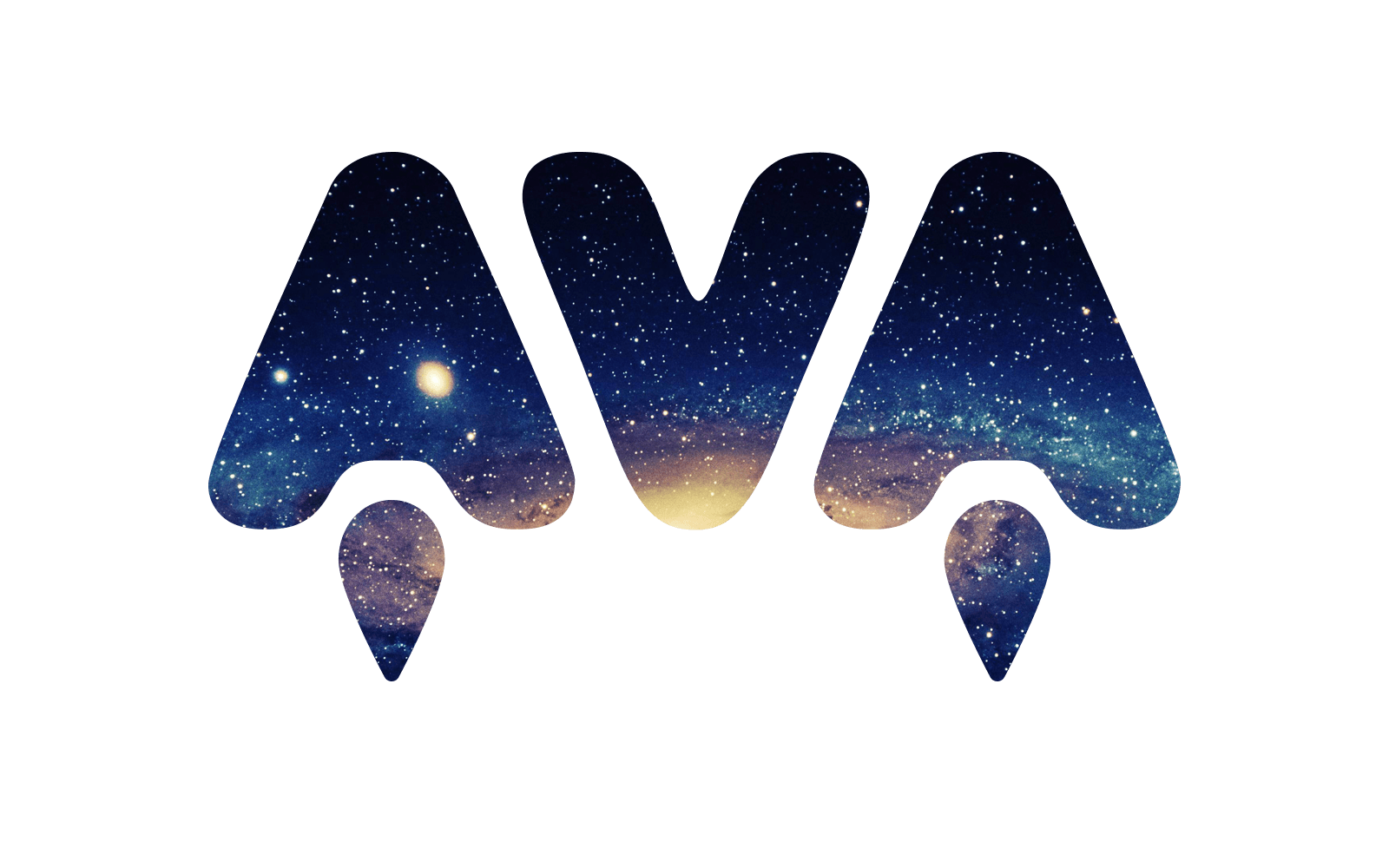
Best for: Isolated, concurrent test execution in performance-sensitive projects
Pros:
- Lightweight, simple syntax, and magic assertion.
- Minimalistic approach and no implicit globals.
Cons:
- Lacks built-in mocking.
- Inconvenient documentation on GitHub in the form of .md files.
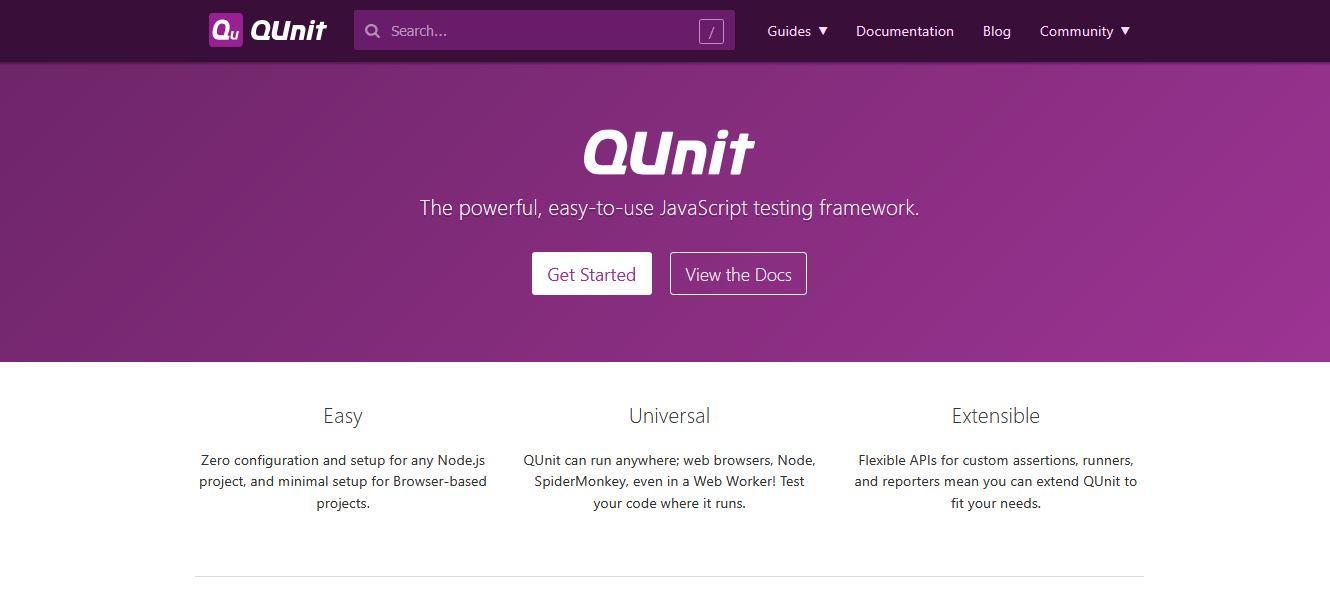
Best for: Testing jQuery and intricate DOM interactions
Pros:
- Simple, easy-to-use, beginner-friendly.
- Test fixtures and custom assertions, reporters, and runners.
Cons:
- Complicated asynchronous testing.
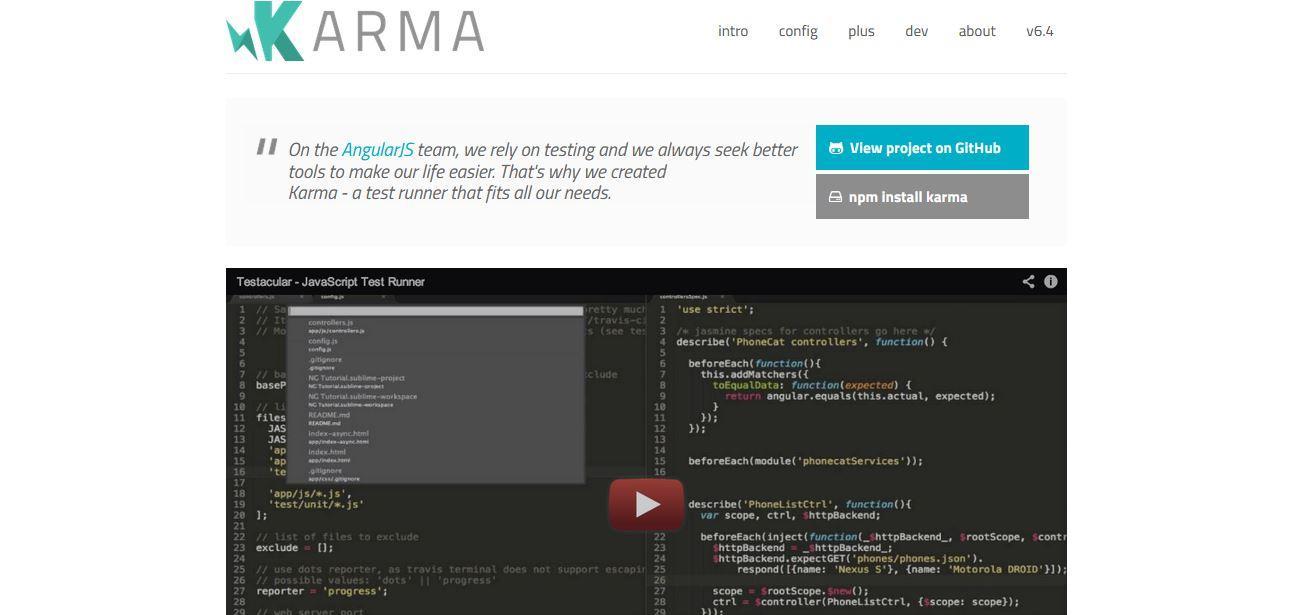
Best for: Real-browser test runs in Angular and cross-browser projects
Pros:
- Support for multiple browsers and legacy browsers.
- Seamless integration with Angular CLI.
Cons:
- Deprecated and will not be accepting any new features or bug fixes.
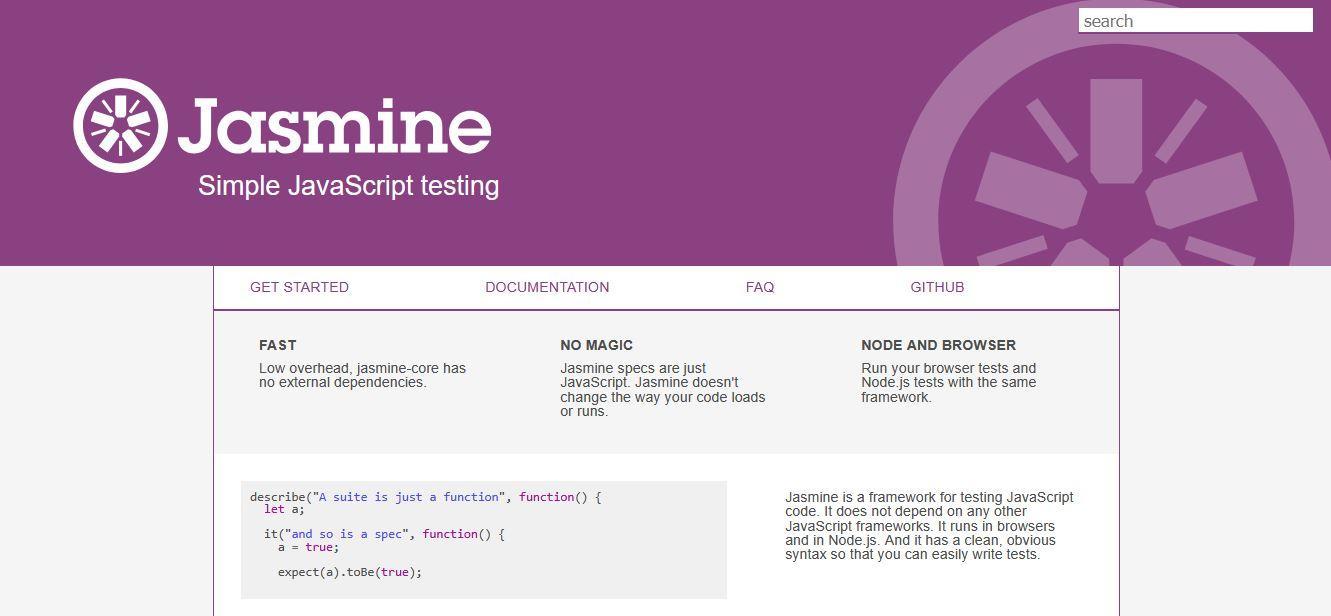
Best for: BDD tests without external dependencies
Pros:
- Simple API with clean, intuitive syntax.
- Built-in assertion methods and spies.
- Highly versatile and do not require DOM support.
Cons:
- Initial configuration can be tricky.
- Difficulties with asynchronous testing.
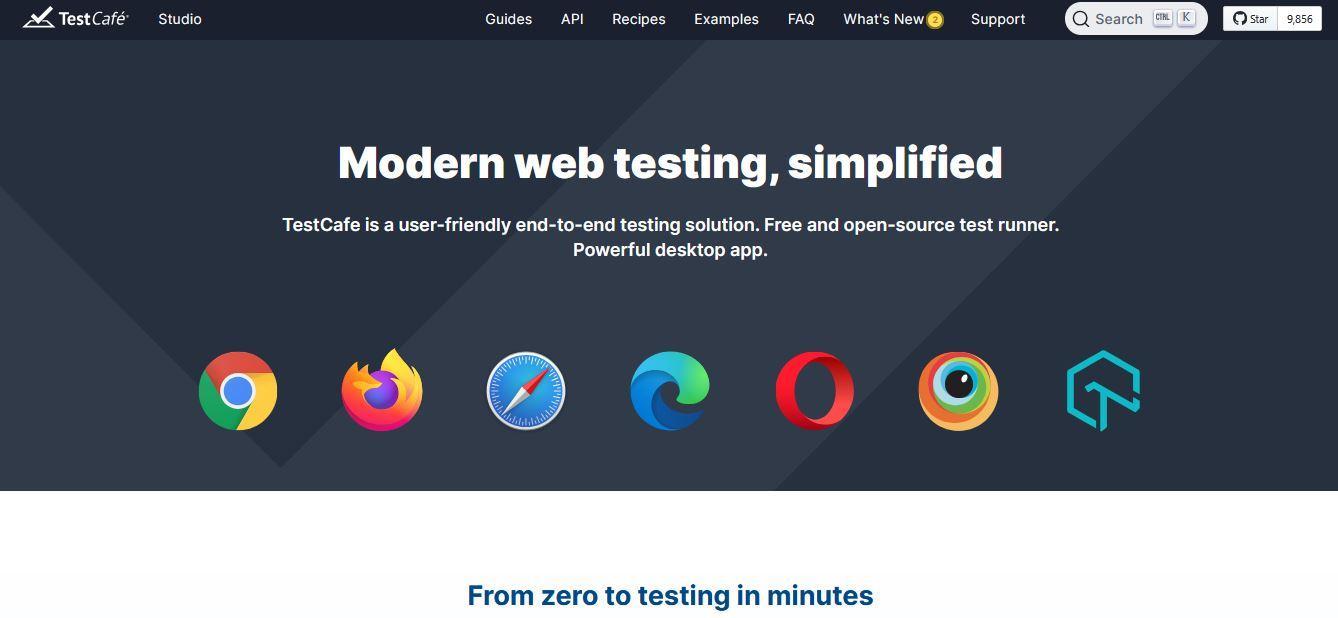
Best for: Testing with minimal setup overhead
Pros:
- Parallel, multi-window, cross-browser testing.
- Live mode for easy debugging.
- Simple setup and easy CI/CD integration—ideal for pipelines that lean on automated unit tests from the start.
Cons:
- Better suited for end-to-end testing rather than unit testing.
- Limited reporting capabilities.
Python developers tend to lean on simplicity and readability—so it makes sense that the most popular testing frameworks follow suit. Whether you're testing fast APIs or complex data pipelines, these tools range from minimal to fully extensible.
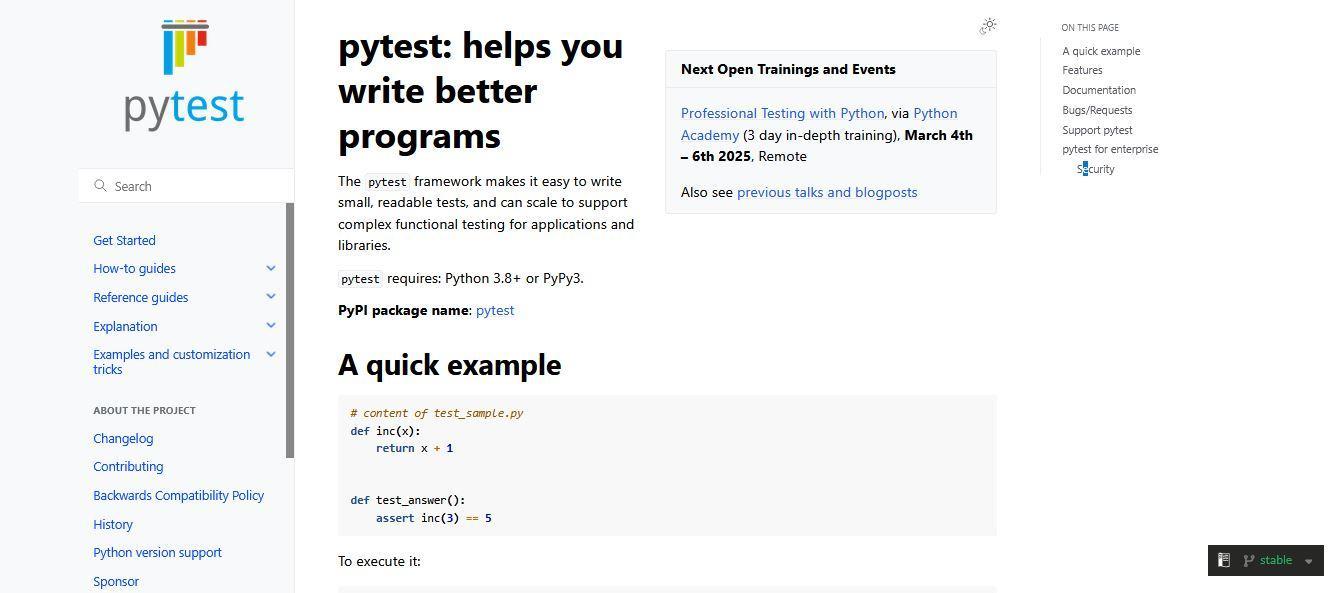
Best for: Advanced Python projects requiring flexibility and deep test coverage
Pros:
- Enhanced test organization and readability with robust fixtures.
- Supports parameterized testing to test different input values without test duplication.
- Extensive plugin support.
- Pytest’s flexibility also makes it great for teams focused on code quality metrics beyond just coverage numbers.
Cons:
- Customization needs a deeper technical understanding and is challenging.
- Complex CI/CD integration.
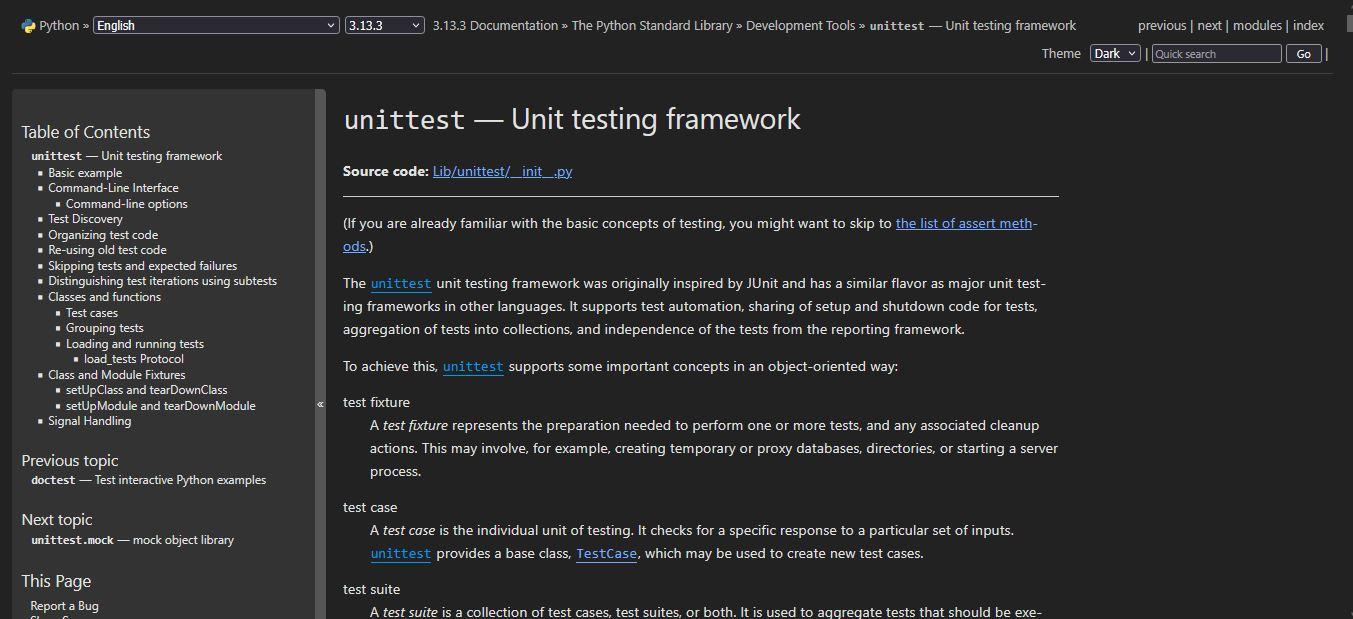
Best for: When a stable, highly compatible, built-in testing framework without external dependencies is needed
Pros:
- Readily available as it is included in the Python standard library.
- Compatible with older Python versions and integrates well with legacy systems.
- Grouping related tests into suites.
Cons:
- Complex setup, less extensible, and inconveniently verbose syntax.
- Manual implementation is required for parameterization.
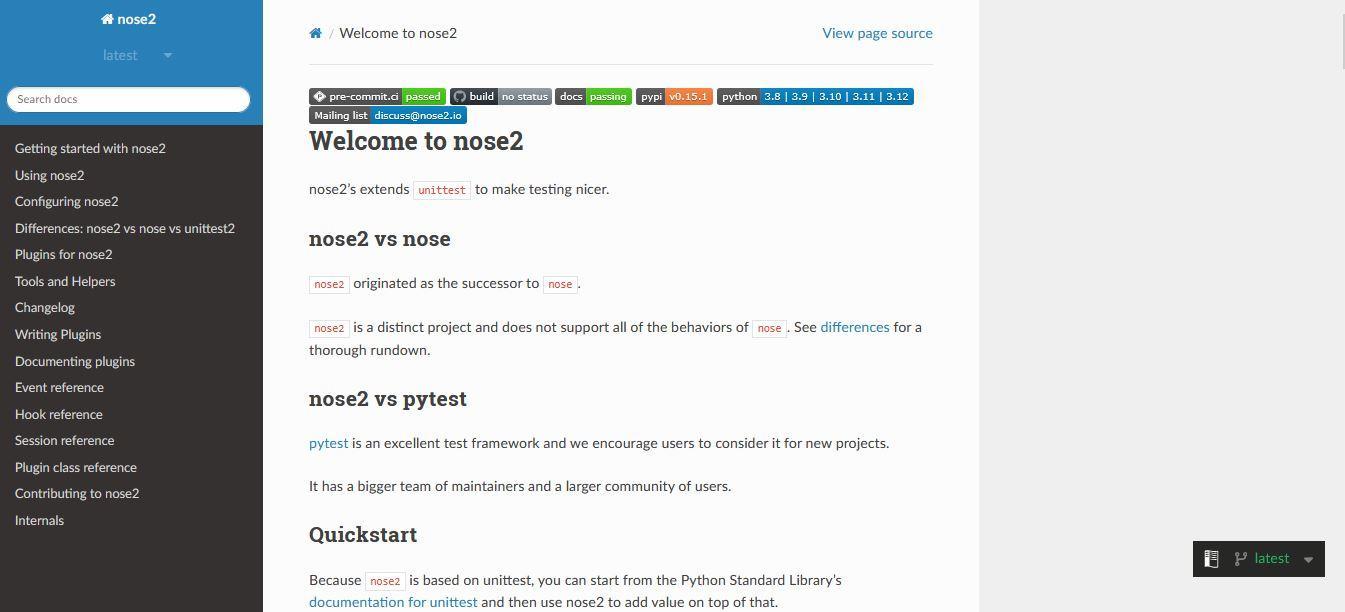
Best for: Teams planning to transition from unit test to a more user-friendly solution with additional features
Pros:
- Better performance with parallel testing.
- Simplified and speedy testing with plugins.
- Extended customization and automatic test discovery.
Cons:
- Lack of active maintenance and comprehensive documentation
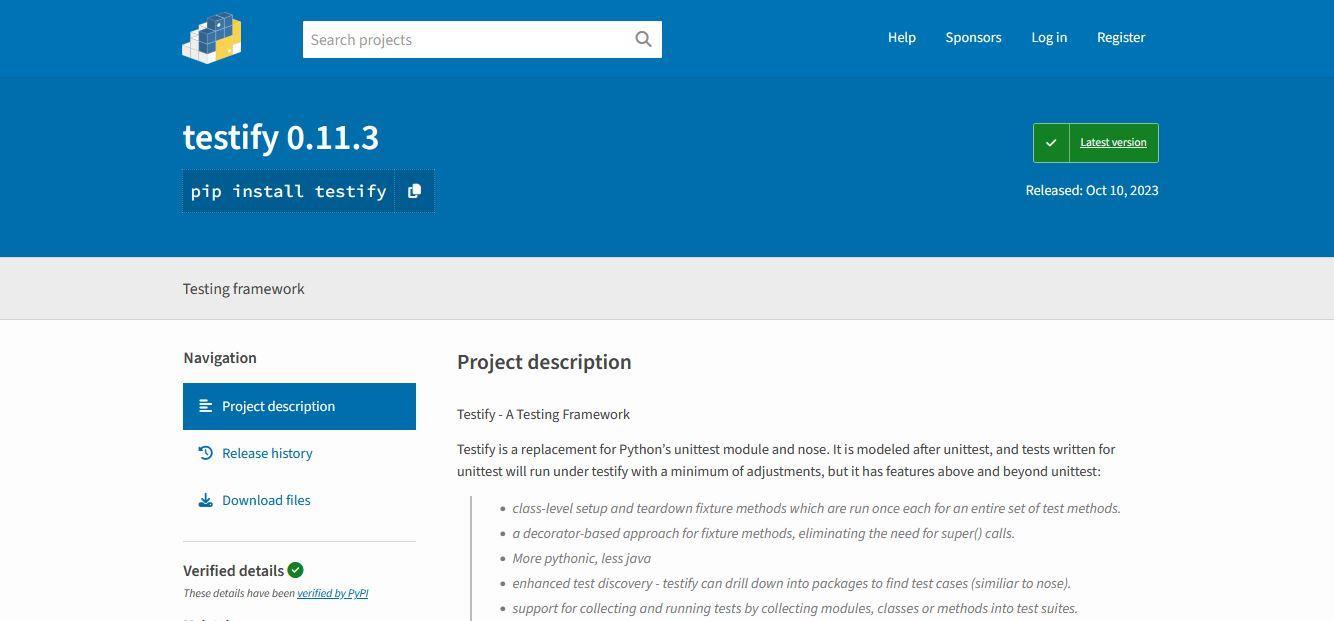
Best for: Developers looking for a modern unit test alternative with clearer syntax
Pros:
- Easy to understand, more Pythonic, less Java syntax.
- Enhanced test discovery capabilities, similar to nose2.
- Rich array of plugins for additional functionality.
Cons:
- Limited documentation.
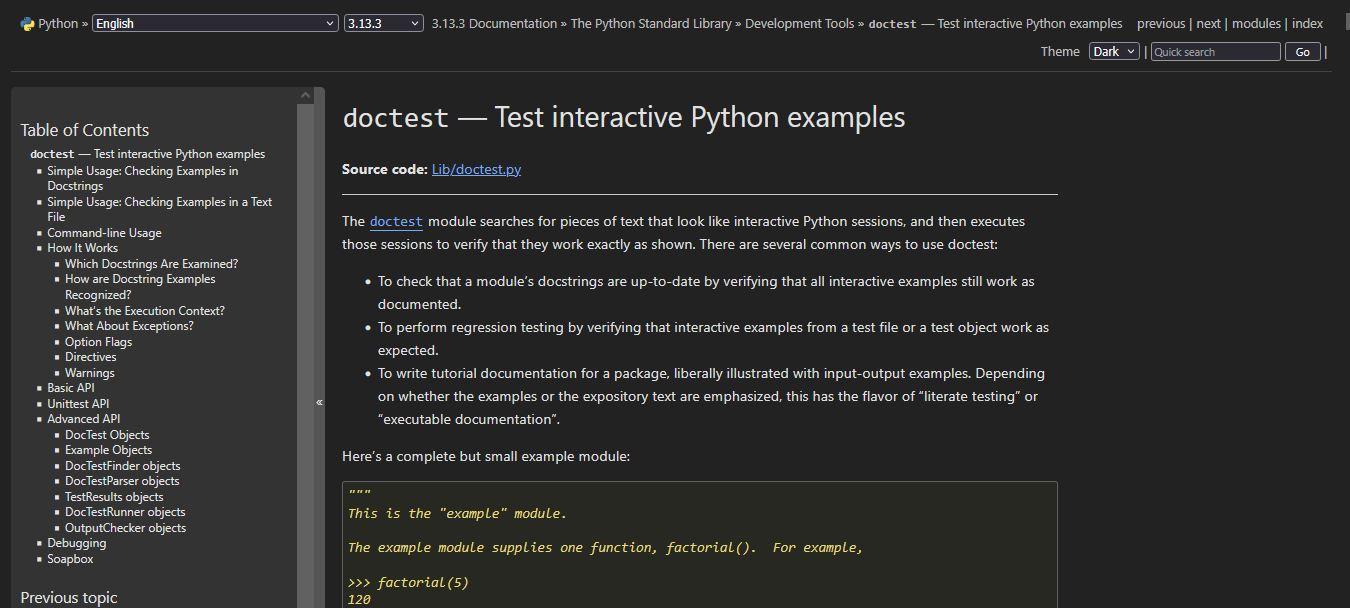
Best for: Small scripts that need spot checks
Pros:
- Zero setup, minimal learning curve, and fewer requirements for extra dependencies.
- Allows writing simple and quick tests alongside the code and performing quick sanity checks.
Cons:
- Lacks hooks, assertions, mocking, or fixtures.
- It’s not suited for comprehensive scans or integration with automated testing tools.
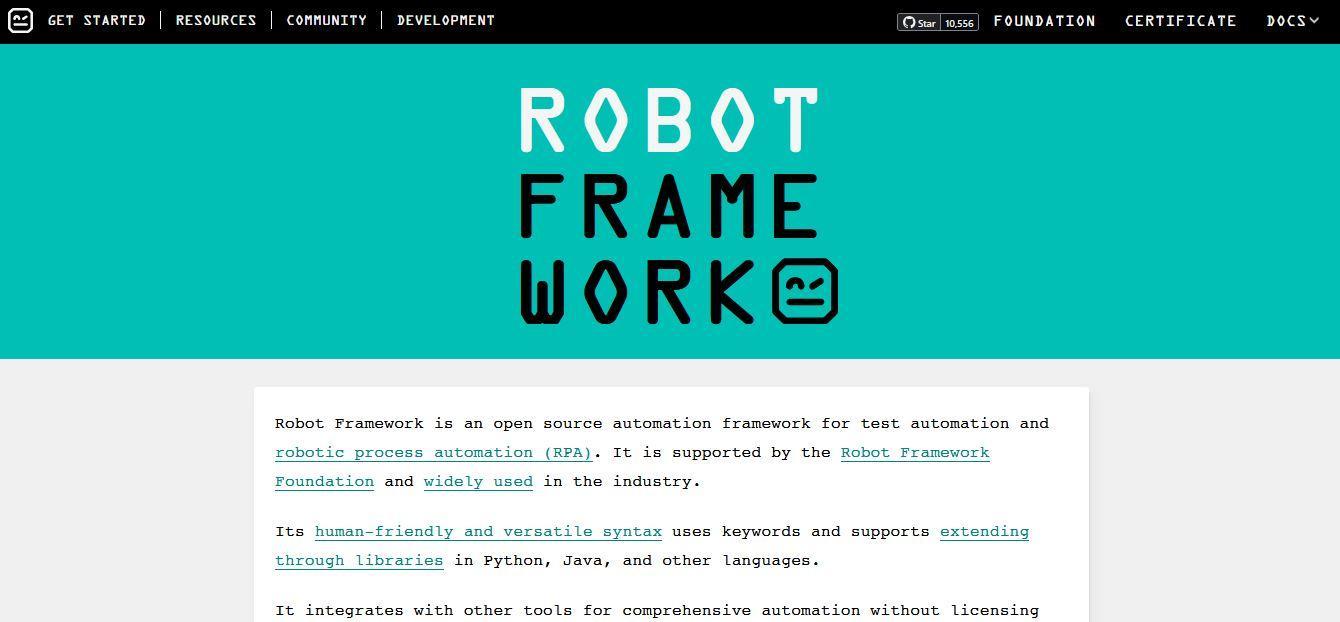
Best for: Keyword-driven testing and non-dev teams. Used more often for acceptance and integration tests, but supports unit tests via libraries.
Pros:
- Uses keywords to allow testers to write readable test cases with a simple syntax.
- Works on different operating systems (Windows, Linux, macOS) and supports programming languages like Python and Java.
- Automatic generation of detailed logs and reports.
Cons:
- Steep learning curve.
- Complex maintenance.
Some frameworks aren’t tied to a specific language—they're designed to be flexible across environments, use cases, and tech stacks. If your team is multi-language or you're working in a polyglot CI/CD setup, these tools might be a better fit.

Best for: Specification-driven testing with multilingual support
Pros:
- Highly maintainable, extensible through plugins, and easy-to-use markdown-based syntax.
- Works with languages, CI/CD tools, and platforms of the developer’s choice.
- Parallel execution and data-driven testing.
Cons:
- Limited community support.
Not every framework is built for mainstream adoption—some are hyper-specialized, experimental, or just underrated. These honorable mentions didn’t make the top list, but they’re worth a look if you have niche requirements or just like testing weird stuff.

Best for: Lightweight modern JavaScript testing
Pros:
- Fast and simple design to achieve higher performance.
- Works in any JavaScript environment like Node.js, Deno, and the browser.
Cons:
- Very limited community and minimal documentation.
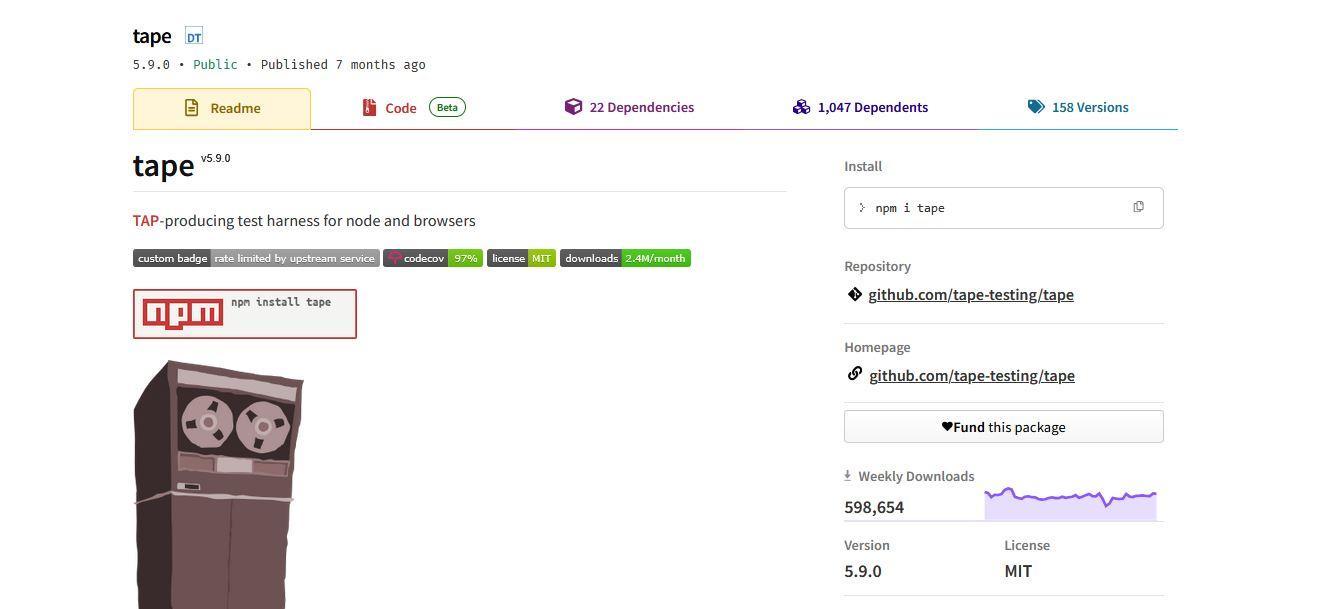
Best for: Simple, Node.js-based tests
Pros:
- Minimal architecture with only the essential features.
- Simple and straightforward.
- Uses TAP (test anything protocol) format for output.
Cons:
- Lack of documentation.
- Limited features.
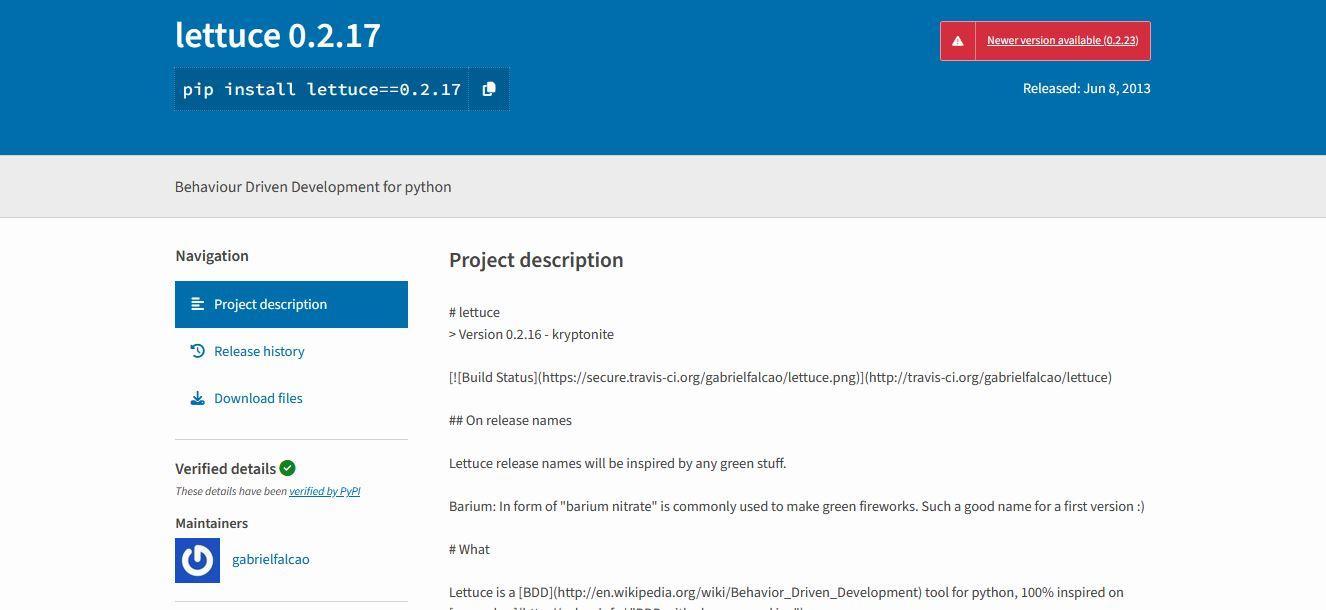
Best for: Gherkin-style tests for Python
Pros:
- Allows writing human-readable test scenarios in natural language.
- Supports BDD.
Cons:
- Lacks feature richness.
- Minimal ongoing support.
- No active maintenance.

Best for: Gherkin with Python
Pros:
- Comprehensive documentation and tutorials.
- Human-readable tests with scenarios written in the Gherkin language. It can also be used to validate zero trust controls when integrated with auth-driven applications.
- Django and Flask integrations are available.
Cons:
- Lacks built-in parallel execution.
- Verbose for unit tests.
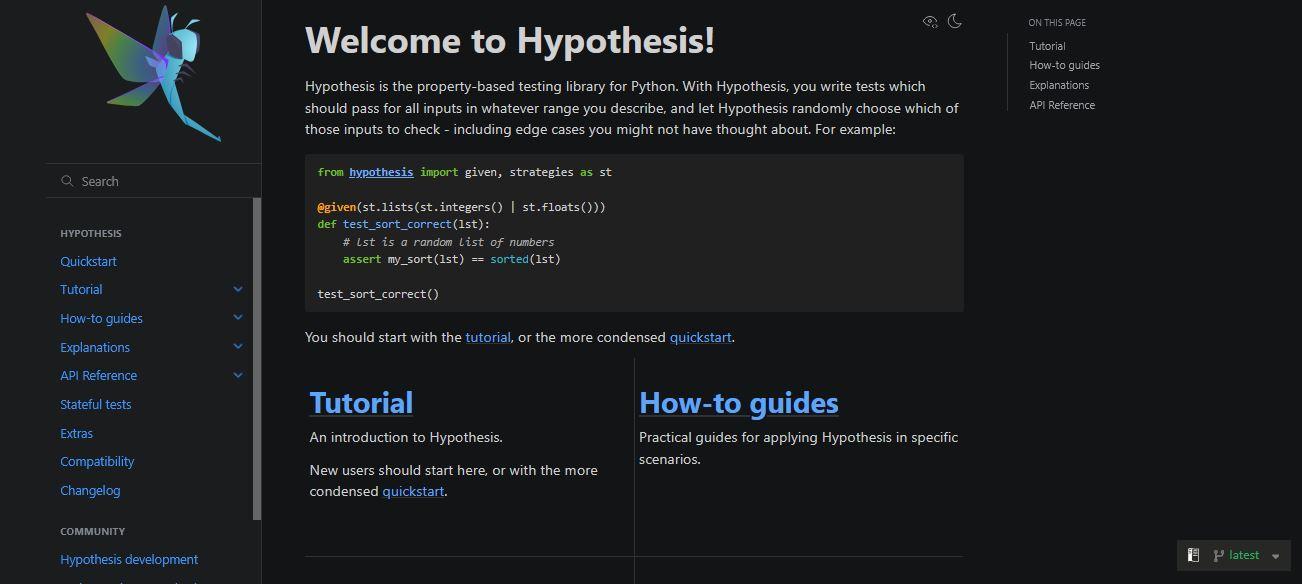
Best for: Property-based testing
Pros:
- Facilitates catching bugs and edge cases that a human tester would miss. Its property-based approach is especially useful in scenarios shaped by strict data security policies.
- Works and is regularly tested on Linux, Windows, and OS X.
- Active community and constant improvements.
Cons:
- Might be overkill for simple unit tests.
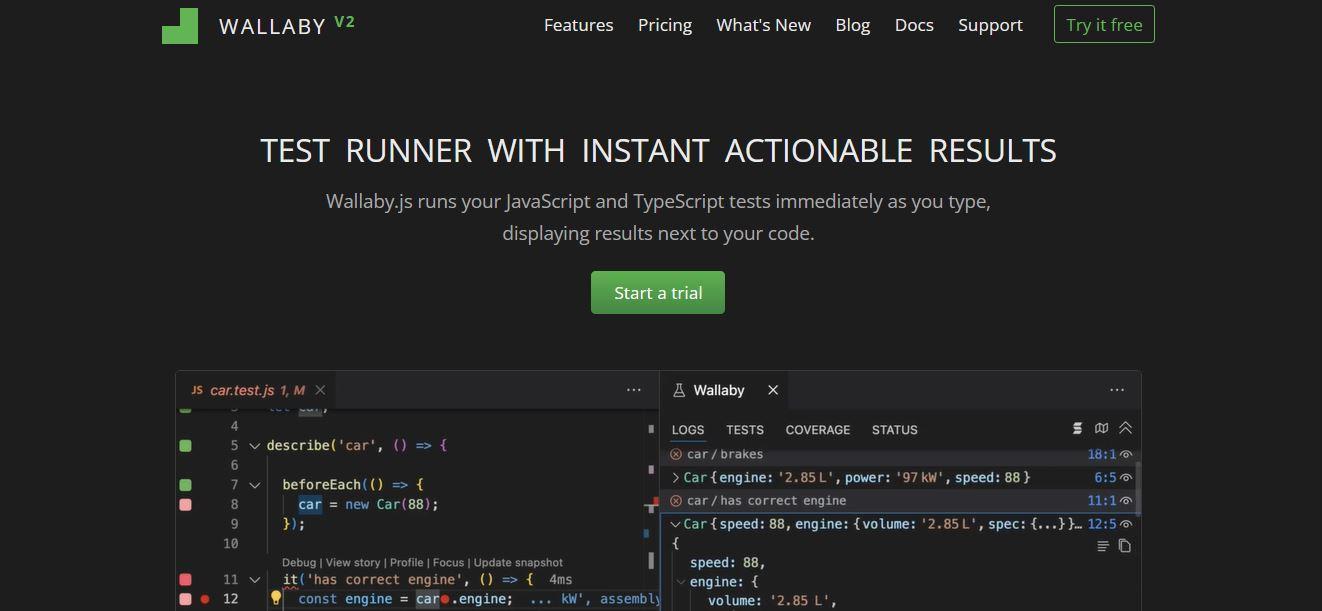
Best for: Real-time testing feedback
Pros:
- Code coverage and test results are displayed in real time on the code editor.
- Inline error reporting and advanced debugging tools.
- Parallel test execution.
Cons:
- Considerably expensive.

Best for: Minimalistic BDD-style assertions
Pros:
- Cross-browser support.
- Node.js ready.
Cons:
- Lacks an active community and updates. The last release was in 2015.
Choose Smarter, Ship Faster
Your testing framework impacts more than just syntax—it defines your team’s speed, stability, and confidence in every release. But even with the right tool, scaling test coverage and maintaining quality is a constant grind. That’s where agentic AI steps in.
Agentic AI takes initiative. EarlyAI uses your existing stack, understands your codebase, and proactively generates tests that stay aligned with your architecture and development flow. It’s built to reduce manual overhead and close the gaps traditional frameworks can’t.
If you’re ready to level up your test strategy, book a demo with EarlyAI and see how agentic AI can help you ship faster without breaking things.